Tutorial: Creating live templates
When developing PHP applications, a commonly repeated task is creating Controller classes and actions in them. This tutorial describes how to create Live Templates to automate this.
Let's start with a Controller class. Controller classes are typically named like BlogController
, and extend some basic Controller
class.
In the Settings dialog (CtrlAlt0S) , select Editor | Live Templates.
Click the
to create a new Live Template. Provide it with an abbreviation, for example,
sc
(standing for Sample Controller), a description, the context in which the template applies, in this case, PHP, and the following text:class $Name$Controller extends Controller { $END$ }
The resulting template should look as follows:
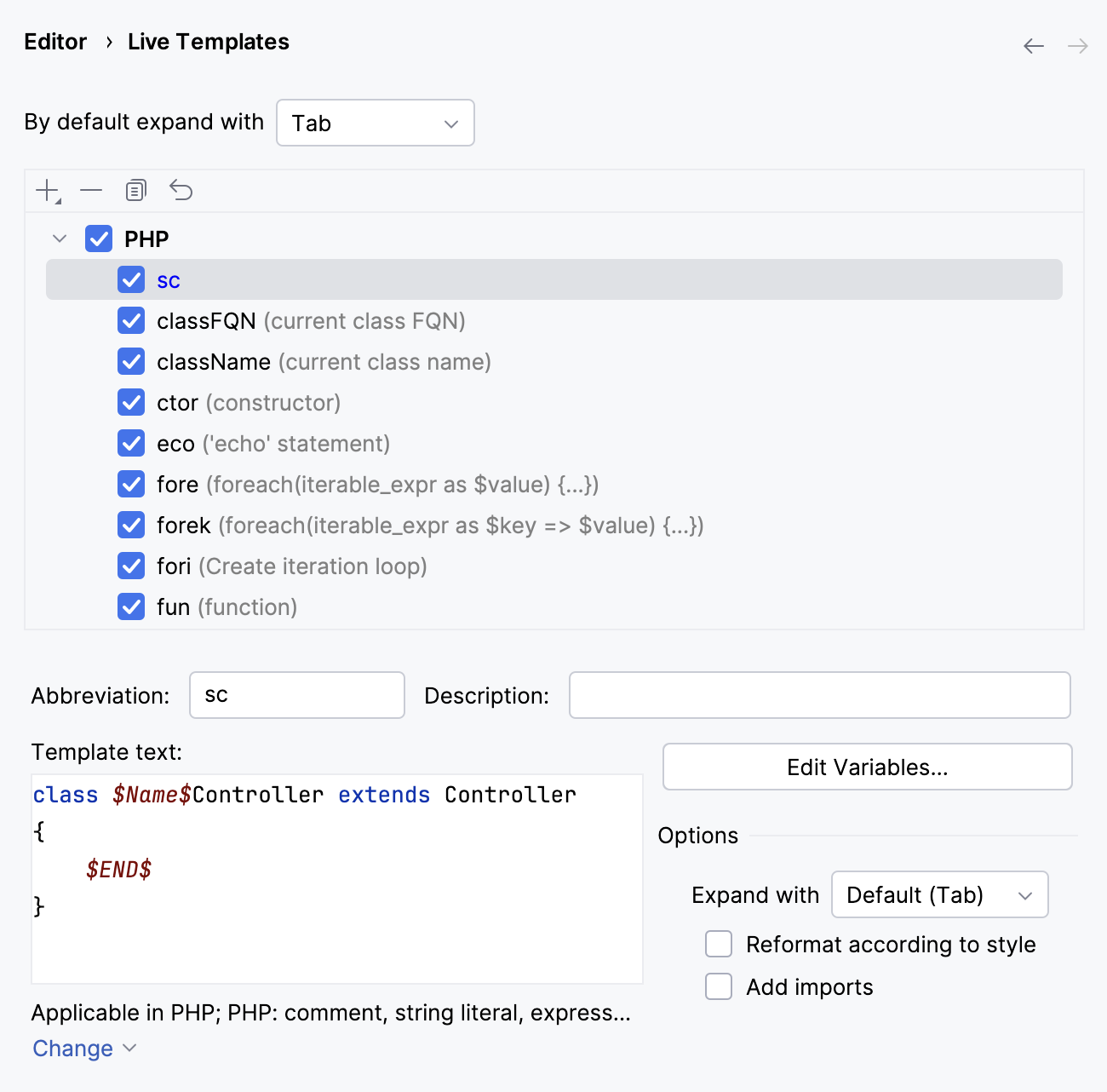
You can now open a php file (or create a new one) and use the created sc
Live Template. Once the template code is expanded, provide the controller's class name and press Tab to move the caret to the $END$
variable's position.
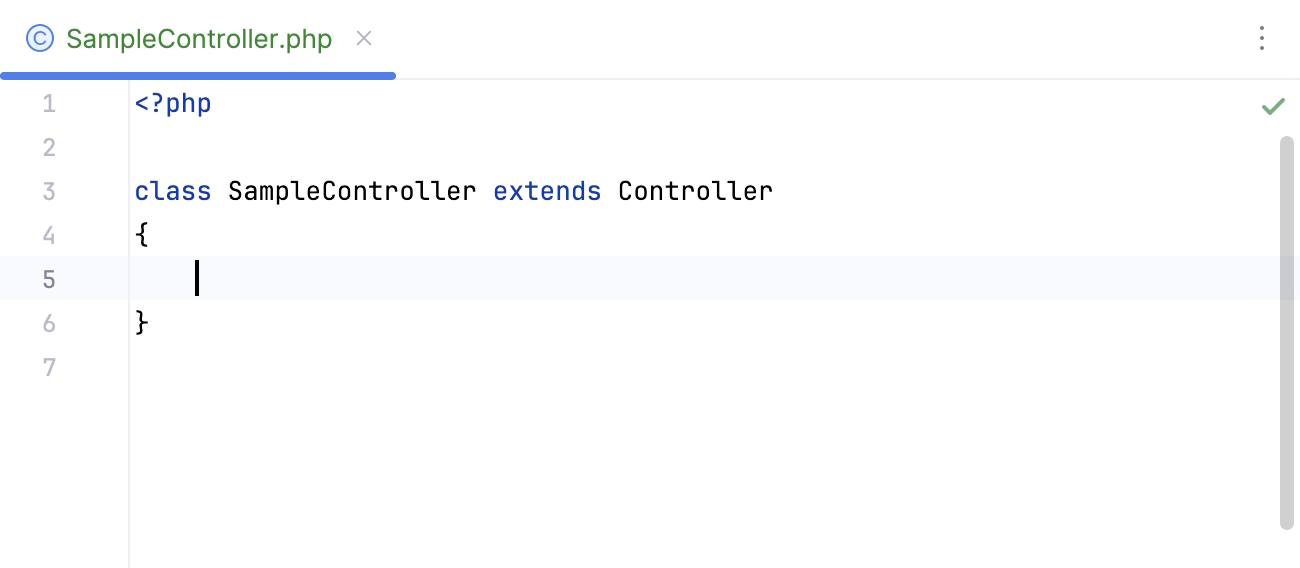
tip
Since each controller class typically resides in its own file, this Sample Controller template could also be implemented as a File Template.
So far, you've used a single $Name$
custom variable and the special $END$
variable provided by PhpStorm. Controller classes are commonly named exactly like the php files they reside in: for example, the MyController.php file will contain the MyController
controller class. With Live Templates, it is possible to have PhpStorm pre-populate the controller name based on the filename.
In the Settings dialog (CtrlAlt0S) , select Editor | Live Templates. Then, select the
sc
template created earlier.Change the
sc
template's text to the following:class $Name$ extends Controller { $END$ }
Click Edit Variables and see that the IDE has recognized
$Name$
as a variable.Configure the
$Name$
variable as follows:Provide a default value and the expression. For our case, use the
fileNameWithoutExtension()
expression, which will return the name of the file without the .php extension.Select the Skip if defined checkbox so that when the expression provides a value, you don't have to provide it yourself when using the live template in the editor.
note
See Live template variables for the full list of available expressions.
When you apply the Live Template, the class will now inherit the filename, and the caret will be positioned inside the class body immediately.
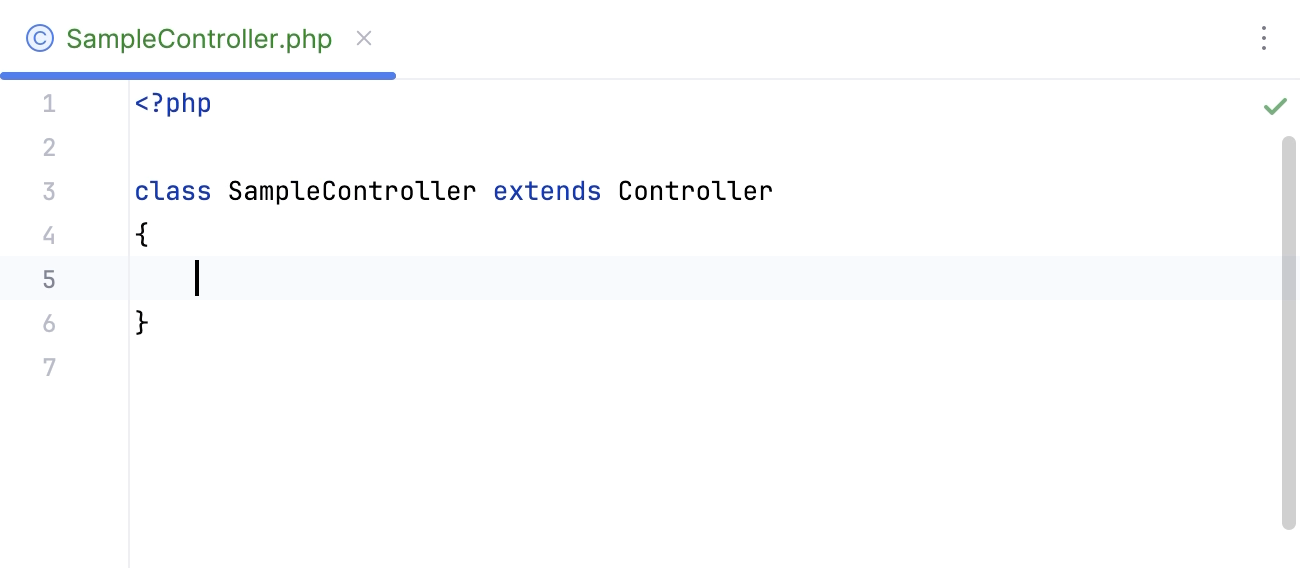
You can use the defined template variables as arguments for expressions. As an example, let's extend the template to include a __toString
method definition.
Change the
sc
template's text to the following:class $NAME$ extends Controller { public $$$PROPERTY$; public function __toString() { return $this->$PROPERTY$; } $END$ }
tip
A doubled dollar sign
$$
is used to escape the PHP's$
dollar sign.Click Edit Variables
Configure the
$PROPERTY$
variable as follows:Provide a default value and the expression. For our case, use the
decapitalize(NAME)
expression, which will return the decapitalized value if the$NAME$
variable, that is, the name of the class without the .php extension.tip
When a template variable is passed into an expression, dollar signs around its name are omitted,
Select the Skip if defined checkbox so that when the expression provides a value, you don't have to provide it yourself when using the live template in the editor.
When you apply the template, the created class will now include a property and a __toString
method definition.
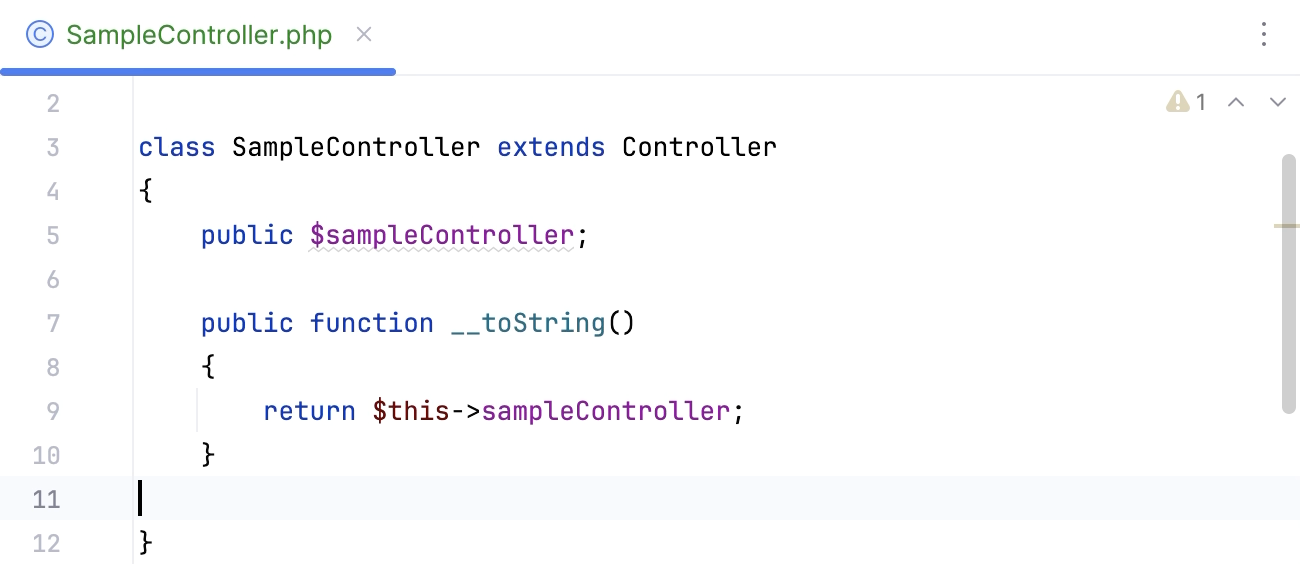
The sc
template was simple enough to be created directly from the Settings dialog. In more complex cases, however, it's probably easier to write code in the editor and then make a template out of it.
Let's create a template for a basic controller action.
In the code editor, write some code just as you do when writing an application.
class SampleController extends Controller { public function indexAction() { return $this->render(''); } }
Select the created
indexAction
method in the editor and select Code | Save as Live Template in the main menu.In the Save as Live Template dialog that opens, notice that the context for this template is automatically set based on the type of the file you were working with in the editor.
Configure the template and add variables by changing the template's text to the following:
public function $Action$Action() { $END$ return $this->render('$Bundle$:$Folder$:$Action$.html.twig'); }
Click Edit Variables and specify the expression for the
$Folder$
variable and the default value for the$Bundle$
variable. Note that the default value must be enclosed in double quotation marks.
Having applied the changes, you can use the sa template to generate a controller action.
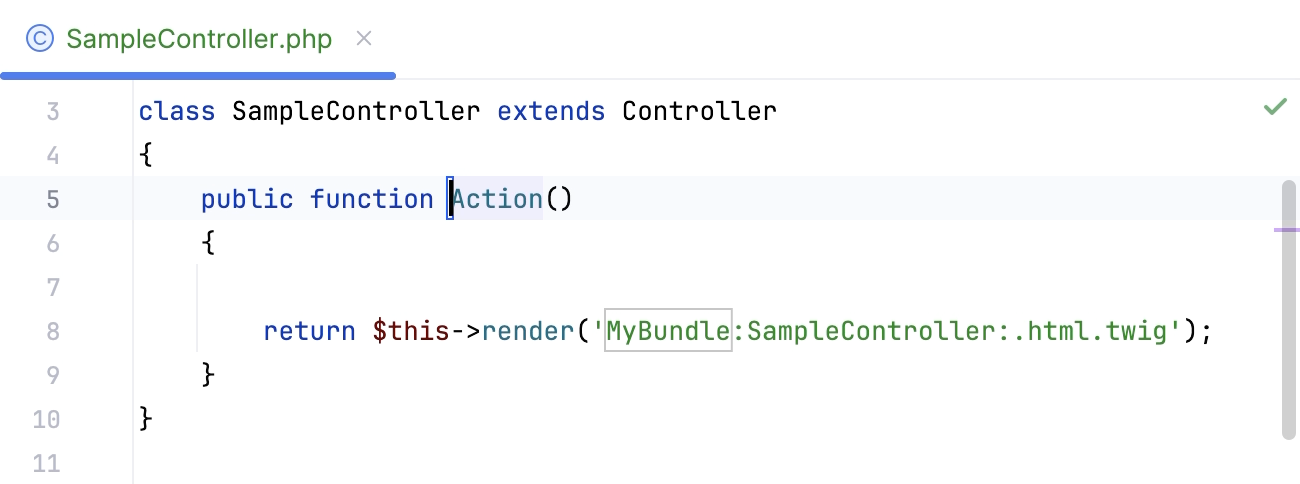
In some cases, it may be useful to "surround" the existing code with a template. As the name implies, Surround Templates let you do just that. They can be created in the exact same way as Live Templates but additionally involve using of the $SELECTION$
variable.
As an example, let's create a Surround Template that wraps the selected code in a try/catch
block and logs the exception.
Create a live template containing the following text (notice that a doubled dollar sign
$$
is used to escape the PHP's$
dollar sign):try { $SELECTION$ } catch ($TYPE$ $$$VARIABLENAME$) { Logger::log($$$VARIABLENAME$); $END$ }
The following variables are used in the template:
$SELECTION$
Denotes the code selected in the editor.
$TYPE$
Serves as a placeholder for the exception type.
$VARIABLENAME$
Serves as a placeholder for the exception variable name.
$END$
Indicates the caret position after the template is expanded.
In the Edit Variables dialog, provide
$TYPE$
with thecomplete()
expression, so that when you expand the template, completion is triggered. Additionally, provide the default values for$TYPE$
and$VARIABLENAME$
enclosed in double quotation marks.
Having applied the changes, you can use the surround template as follows: select some code, press CtrlAlt0J, and select the newly created template.
As a result, the code is wrapped in the template, completion is triggered on the exception type, and the exception variable is named $exception
by default. You can pick the correct type and press Tab to end up in the catch
body, right underneath the logging statement.
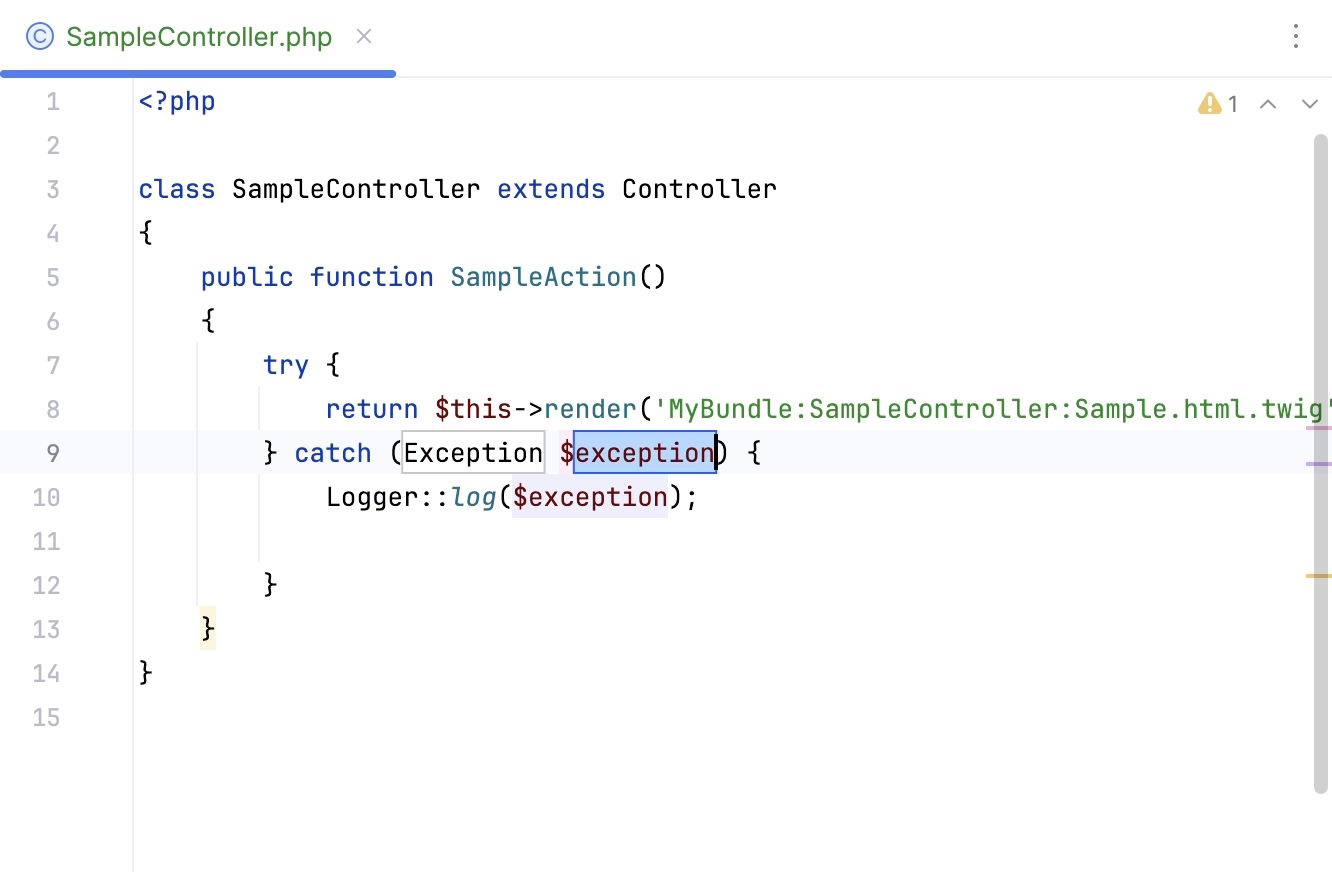
Thanks for your feedback!